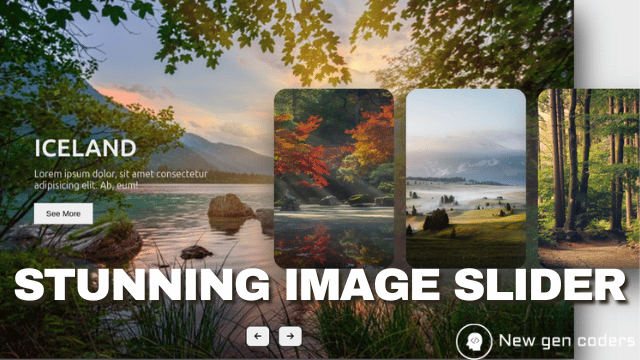
Travel Destinations Image Slider
- Nov 13, 2024
- 26
To create aesthetically beautiful 3D hover effects for cards that offer a fun and interactive experience for users.
How to Design:
HTML Setup: Make a card layout with character, title, and cover picture placeholders.
Define the card's size, placement, and hover effects using CSS styling. For fluid animations, include transitions.
Interactive Effects: Create a 3D depth simulation by using perspective and transform parameters. Use gradients and layers to enhance the visual effect.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>3D Card Hover Effect | code_wars_official</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<a href="#">
<div class="card">
<div class="wrapper">
<img src="dark_rider-cover.jpg" class="cover-image" />
</div>
<img src="dark_rider-title.png" class="title" />
<img src="dark_rider-character.webp" class="character" />
</div>
</a>
<a href="#">
<div class="card">
<div class="wrapper">
<img src="force_mage-cover.jpg" class="cover-image" />
</div>
<img src="force_mage-title.png" class="title" />
<img src="force_mage-character.webp" class="character" />
</div>
</a>
</body>
</html>
:root {
--card-height: 300px;
--card-width: calc(var(--card-height) / 1.5);
}
*{
box-sizing: border-box;
}
body {
width: 100vw;
height: 100vh;
margin: 0;
display: flex;
justify-content: center;
align-items: center;
background: white;
}
.card {
width: var(--card-width);
height: var(--card-height);
position: relative;
display: flex;
justify-content: center;
align-items: flex-end;
padding: 0 36px;
perspective: 2500px;
margin: 0 50px;
border-radius: 5px;
}
.cover-image{
width: 100%;
height: 100%;
object-fit: cover;
border-radius: 5px;
}
.wrapper{
transition: all 0.5s;
position: absolute;
width: 100%;
z-index: -1;
border-radius: 5px;
}
.card:hover .wrapper{
transform: perspective(900px) translateY(-5%) rotateX(25deg) translateZ(0);
box-shadow: 2px 35px 32px -8px rgba(0, 0, 0, 0.75);
-webkit-box-shadow:2px 35px 32px -8px rgba(0, 0, 0, 0.75);
-moz-box-shadow:2px 35px 32px -8px rgba(0, 0, 0, 0.75);
border-radius: 5px;
}
.wrapper::before,
.wrapper::after{
content: "";
opacity: 0;
width: 100%;
height: 80px;
transition: all 0.5s;
position: absolute;
left: 0;
border-radius: 5px;
}
.wrapper::before{
top: 0;
height: 100%;
background-image: linear-gradient(
to top,
transparent 46%,
rgba(12, 13, 19, 0.5)68%,
rgba(12, 13, 19) 97%
);
border-radius: 5px;
}
.wrapper::after{
bottom: 0;
opacity: 1;
background-image: linear-gradient(
to bottom,
transparent 46%,
rgba(12, 13, 19, 0.5) 68%,
rgba(12, 13, 19)97%
);
border-radius: 5px;
}
.card:hover .wrapper::before,
.wrapper::after{
opacity: 1;
}
.card:hover .wrapper::after{
height: 120px;
}
.title {
width: 100%;
transition: transform 0.5s;
}
.card:hover .title {
transform: translate3d(0%, -50px, 100px);
}
.character {
width: 100%;
opacity: 0;
transition: all 0.5s;
position: absolute;
z-index: -1;
}
.card:hover .character {
opacity: 1;
transform: translate3d(0%, -30%, 100px);
}
Leave Comments