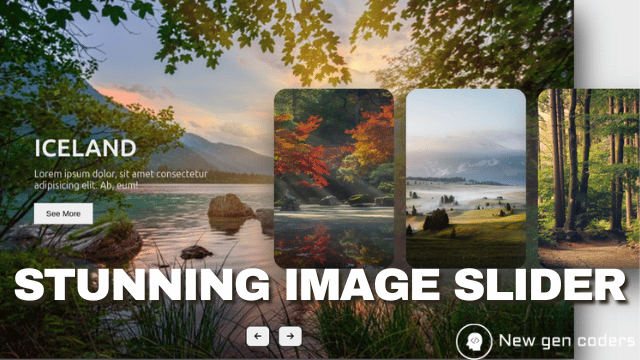
Travel Destinations Image Slider
- Nov 13, 2024
- 26
To create aesthetically beautiful 3D watch designs that offer a fun and interactive experience for users.
How to Design:
React components were used to organise the watch design so that the code was modular and reusable.
Use CSS to style the elements, utilising responsive design concepts, gradients, and shadows.
To replicate depth and lifelike movement, 3D transformations and animations were used.
Real-time ticking and interactive hover effects were implemented using React's state and lifecycle methods, sometimes known as hooks. The fact that React was used in the project is appropriately reflected in this upgraded version.
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="UTF-8">
<title>React Clock Concept</title>
<link rel="stylesheet" href="./style.css">
</head>
<body>
<!-- partial:index.partial.html -->
<div id="root"></div>
<!-- partial -->
<script src='https://codepen.io/yoann-b/pen/qBeVQoB.js'></script><script type="module" src="./script.js"></script>
</body>
</html>
@import url("https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap");
* {
box-sizing: border-box;
font-family: "Quicksand", sans-serif;
}
body {
--digit-active-color: #35c4ff;
--digit-color: #35c2ff7c;
--digit-width: 55px;
--digit-height: 10px;
--main-color: #0d0d0c;
--seconday-color: #1c1c1c;
--main-gradient: linear-gradient(90deg, #35c4ff 0%, #b24eff 100%);
margin: 0;
padding: 0;
background-color: var(--main-color);
color: white;
background-size: cover;
background-image: url("data:image/svg+xml,%3csvg xmlns='http://www.w3.org/2000/svg' version='1.1' xmlns:xlink='http://www.w3.org/1999/xlink' xmlns:svgjs='http://svgjs.dev/svgjs' width='800' height='560' preserveAspectRatio='none' viewBox='0 0 800 560'%3e%3cg mask='url(%26quot%3b%23SvgjsMask1059%26quot%3b)' fill='none'%3e%3cuse xlink:href='%23SvgjsSymbol1066' x='0' y='0'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsSymbol1066' x='720' y='0'%3e%3c/use%3e%3c/g%3e%3cdefs%3e%3cmask id='SvgjsMask1059'%3e%3crect width='800' height='560' fill='white'%3e%3c/rect%3e%3c/mask%3e%3cpath d='M-1 0 a1 1 0 1 0 2 0 a1 1 0 1 0 -2 0z' id='SvgjsPath1065'%3e%3c/path%3e%3cpath d='M-3 0 a3 3 0 1 0 6 0 a3 3 0 1 0 -6 0z' id='SvgjsPath1063'%3e%3c/path%3e%3cpath d='M-5 0 a5 5 0 1 0 10 0 a5 5 0 1 0 -10 0z' id='SvgjsPath1062'%3e%3c/path%3e%3cpath d='M2 -2 L-2 2z' id='SvgjsPath1060'%3e%3c/path%3e%3cpath d='M6 -6 L-6 6z' id='SvgjsPath1061'%3e%3c/path%3e%3cpath d='M30 -30 L-30 30z' id='SvgjsPath1064'%3e%3c/path%3e%3c/defs%3e%3csymbol id='SvgjsSymbol1066'%3e%3cuse xlink:href='%23SvgjsPath1060' x='30' y='30' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='30' y='90' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='30' y='150' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='30' y='210' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='30' y='270' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='30' y='330' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1064' x='30' y='390' stroke='rgba(53%2c 196%2c 255%2c 0.3)' stroke-width='3'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='30' y='450' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='30' y='510' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='30' y='570' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='90' y='30' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='90' y='90' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='90' y='150' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='90' y='210' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1065' x='90' y='270' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='90' y='330' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='90' y='390' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='90' y='450' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='90' y='510' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='90' y='570' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='150' y='30' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='150' y='90' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='150' y='150' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='150' y='210' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='150' y='270' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='150' y='330' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='150' y='390' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='150' y='450' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='150' y='510' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='150' y='570' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='210' y='30' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='210' y='90' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='210' y='150' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='210' y='210' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='210' y='270' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='210' y='330' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='210' y='390' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='210' y='450' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='210' y='510' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='210' y='570' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='270' y='30' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='270' y='90' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='270' y='150' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='270' y='210' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='270' y='270' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='270' y='330' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='270' y='390' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='270' y='450' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='270' y='510' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='270' y='570' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='330' y='30' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='330' y='90' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='330' y='150' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1065' x='330' y='210' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1064' x='330' y='270' stroke='rgba(53%2c 196%2c 255%2c 0.3)' stroke-width='3'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='330' y='330' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='330' y='390' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='330' y='450' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1065' x='330' y='510' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='330' y='570' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='390' y='30' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='390' y='90' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1065' x='390' y='150' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='390' y='210' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='390' y='270' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='390' y='330' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='390' y='390' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='390' y='450' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='390' y='510' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='390' y='570' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='450' y='30' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='450' y='90' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='450' y='150' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='450' y='210' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='450' y='270' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1065' x='450' y='330' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='450' y='390' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='450' y='450' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='450' y='510' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='450' y='570' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='510' y='30' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='510' y='90' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='510' y='150' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1064' x='510' y='210' stroke='rgba(53%2c 196%2c 255%2c 0.3)' stroke-width='3'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='510' y='270' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='510' y='330' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='510' y='390' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='510' y='450' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='510' y='510' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='510' y='570' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1065' x='570' y='30' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='570' y='90' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='570' y='150' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='570' y='210' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='570' y='270' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='570' y='330' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='570' y='390' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='570' y='450' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='570' y='510' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='570' y='570' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='630' y='30' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='630' y='90' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='630' y='150' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='630' y='210' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='630' y='270' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='630' y='330' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1065' x='630' y='390' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='630' y='450' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='630' y='510' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1065' x='630' y='570' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='690' y='30' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='690' y='90' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1062' x='690' y='150' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1065' x='690' y='210' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1061' x='690' y='270' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='690' y='330' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1063' x='690' y='390' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='690' y='450' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='690' y='510' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3cuse xlink:href='%23SvgjsPath1060' x='690' y='570' stroke='rgba(53%2c 196%2c 255%2c 0.3)'%3e%3c/use%3e%3c/symbol%3e%3c/svg%3e");
}
main {
min-height: 100vh;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
gap: 20px;
}
ul {
list-style: none;
margin: 0;
padding: 0;
}
.Clock {
width: var(--clock-size);
height: var(--clock-size);
transform: translateY(calc(var(--clock-size) / 4));
position: relative;
overflow: hidden;
clip-path: circle(30% at 50% 0);
border: 2px solid #35c4ff;
border-radius: 50%;
}
.Clock > li {
position: absolute;
inset: 0;
}
.Clock > li:nth-child(1) {
transform: translateY(20px);
}
.Clock > li:nth-child(2) {
transform: translateY(50px);
}
.Clock > li:nth-child(3) {
transform: translateY(80px);
}
.ClockCircle {
position: relative;
border-radius: 50%;
transform-origin: center;
transition: transform 0.5s cubic-bezier(0.175, 0.885, 0.32, 1.275);
}
.ClockCircleNumber {
position: absolute;
text-align: center;
border-radius: 50%;
font-weight: bold;
opacity: 0.5;
transform: translate(-50%, -50%);
font-size: 1em;
transition: all 0.5s cubic-bezier(0.175, 0.885, 0.32, 1.275);
}
.ClockCircleNumber.selected {
opacity: 1;
font-size: 1.5em;
}
import React from "https://esm.sh/react";
import ReactDOM from "https://esm.sh/react-dom";
function getTimeDigits() {
const now = new Date();
const digits = {
hours: now.getHours(),
minutes: now.getMinutes(),
seconds: now.getSeconds() };
return digits;
}
function CircleNumbers({ number, angleIndex, size }) {
const stepAngle = 360 / number;
const [angle, setAngle] = React.useState(angleIndex * stepAngle);
React.useEffect(() => {
setAngle(angle => angleIndex * stepAngle);
}, [angleIndex]);
const radius = size / 2;
const center = size / 2;
const numberSize = size * 0.1;
const numbers = Array.from({ length: number }, (_, i) =>
String(i).padStart(2, "0"));
return /*#__PURE__*/(
React.createElement("div", {
className: "ClockCircle",
style: {
width: `${size}px`,
height: `${size}px`,
transform: `rotate(${angle}deg)` } },
numbers.map((num, index) => {
const a = index / number * 2 * Math.PI;
const x = center + radius * Math.sin(a);
const y = center - radius * Math.cos(a);
return /*#__PURE__*/(
React.createElement("div", {
key: num,
className: `ClockCircleNumber ${
Math.abs(angleIndex) === parseInt(num) ? "selected" : ""
}`,
style: {
width: `${numberSize}px`,
height: `${numberSize}px`,
lineHeight: `${numberSize}px`,
left: `${x}px`,
top: `${y}px`,
transform: `translate(-50%, -50%) rotate(${-angle}deg)` } },
num));
})));
}
function App() {
React.useEffect(() => {
if (typeof addVideoLinks === "function") {
addVideoLinks("7443865247158488342", "Tyex-a26AvA", "#35c4ff");
}
}, []);
const [hours, setHours] = React.useState(null);
const [minutes, setMinutes] = React.useState(null);
const [seconds, setSeconds] = React.useState(null);
React.useEffect(() => {
const interval = setInterval(() => {
const { hours: h, minutes: m, seconds: s } = getTimeDigits();
setHours(h);
setMinutes(m);
setSeconds(s);
}, 1000);
}, []);
const clockSize = 800;
return /*#__PURE__*/(
React.createElement("main", null, /*#__PURE__*/
React.createElement("ul", { className: "Clock", style: { "--clock-size": `${clockSize}px` } }, /*#__PURE__*/
React.createElement("li", null,
hours !== null && /*#__PURE__*/
React.createElement(CircleNumbers, {
number: 24,
angleIndex: 0 - hours,
size: clockSize })), /*#__PURE__*/
React.createElement("li", null,
minutes !== null && /*#__PURE__*/
React.createElement(CircleNumbers, {
number: 60,
angleIndex: 0 - minutes,
size: clockSize })), /*#__PURE__*/
React.createElement("li", null,
seconds !== null && /*#__PURE__*/
React.createElement(CircleNumbers, {
number: 60,
angleIndex: 0 - seconds,
size: clockSize })))));
}
ReactDOM.render( /*#__PURE__*/React.createElement(App, null), document.getElementById("root"));
Leave Comments