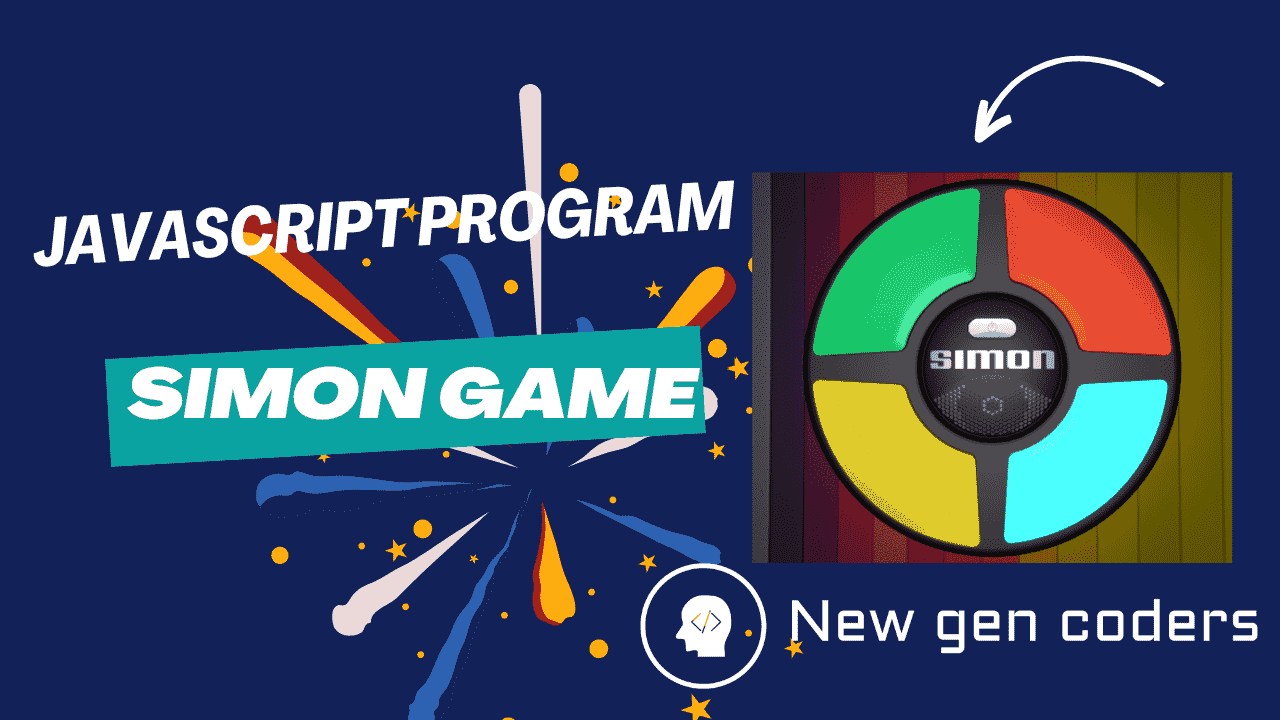
Simon Says Game.
- Jul 21, 2024
- 35
A Simon game is a simple game or brain teaser game in which the player has to repeat the light sequences that the game produces. This is a great project for beginners to learn the core programming concepts of JavaScript. How to declare or use variables in JavaScript to store game states and sequences. How to create functions or use them to handle game logic and respond to user actions.
The game works by lighting a series of game sequence buttons in random order, which the player has to repeat. Once the player successfully repeats the button, a new sequence will be added to the game to make the sequence even more difficult, and if the player does any step wrong, then the game will end and the Game Over message will be shown, the game resets, and the sequence starts over.
Simon game JavaScript involves creating arrays to store the sequence of button presses. Event listeners to detect when the player presses the button and to compare the user sequence or the game sequence This is great for practicing arrays and functions in JavaScript.
Learning how to make a Simon game project in JavaScript is a great way to have fun and improve your skills while learning a lot of programming skills and concepts.
HTML/CSS Fundamentals, JavaScript Basics, Event Handling, DOM Manipulation, Timers and Delays, Arrays and Objects, Game Logic.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>SIMON GAME</title>
<style></style>
</head>
<body>
<h1>Simon Says Game</h1>
<h2 class="highscore">HighScore = 0</h2>
<h2 class="h2">Press any key to start the game</h2>
<div class="btn-container">
<div class="line-one">
<div class="btn red" type="button" id="red"></div>
<div class="btn green" type="button" id="green"></div>
</div>
<div class="line-two">
<div class="btn yellow" type="button" id="yellow"></div>
<div class="btn purple" type="button" id="purple"></div>
</div>
</div>
body {
text-align: center;
}
.red {
background-color: red;
}
.yellow {
background-color: yellow;
}
.green {
background-color: green;
}
.purple {
background-color: purple;
}
.btn {
height: 100px;
width: 100px;
margin-bottom: 10px;
border-radius: 20%;
border: 2px solid black;
}
.btn-container {
display: flex;
gap: 10px;
justify-content: center;
}
.userflash {
background-color: black;
}
.flash {
background-color: white;
}
let gameseq = [];
let userseq = [];
let btns = ["yellow", "red", "green", "purple"];
let started = false;
let level = 0;
let high = 0;
let highscore = document.querySelector(".highscore");
let h2 = document.querySelector(".h2");
let body = document.querySelector("body");
// Event listener for pressing any key to start the game
document.addEventListener("keypress", function () {
if (started == false) {
console.log("Game started");
started = true;
levelup();
}
});
// Function for game flash
function gameflash(btn) {
btn.classList.add("flash");
setTimeout(function () {
btn.classList.remove("flash");
}, 250);
}
// Function for user flash after pressing
function userflash(btn) {
btn.classList.add("userflash");
setTimeout(function () {
btn.classList.remove("userflash");
}, 250);
}
// Function to level up in the game
function levelup() {
userseq = [];
level++;
// Update high score
if (level > high) {
high++;
}
h2.innerText = `Level ${level}`;
highscore.innerText = `HighScore = ${high}`;
let randIdx = Math.floor(Math.random() * 4);
let randColor = btns[randIdx];
let randBtn = document.querySelector(`.${randColor}`);
gameflash(randBtn);
gameseq.push(randColor);
console.log(gameseq);
}
// Check answer
function checkAns(idx) {
console.log("Current level:", level);
if (userseq[idx] === gameseq[idx]) {
console.log("Same Value!!");
if (userseq.length === gameseq.length) {
setTimeout(levelup, 1000);
}
} else {
h2.innerHTML = `Game Over!! Your Score is <b>${level}</b> <br> Press any key to restart!`;
reset();
body.classList.add("red");
setTimeout(function () {
body.classList.remove("red");
}, 200);
}
}
// Function for button flash on user press
function btnpress() {
console.log(this);
userflash(this);
let userColor = this.getAttribute("id");
console.log(userColor);
userseq.push(userColor);
console.log(userseq);
checkAns(userseq.length - 1);
}
let allbtn = document.querySelectorAll(".btn");
for (let btn of allbtn) {
btn.addEventListener("click", btnpress);
}
// Function to reset the game after game over
function reset() {
userseq = [];
gameseq = [];
level = 0;
started = false;
}
Leave Comments