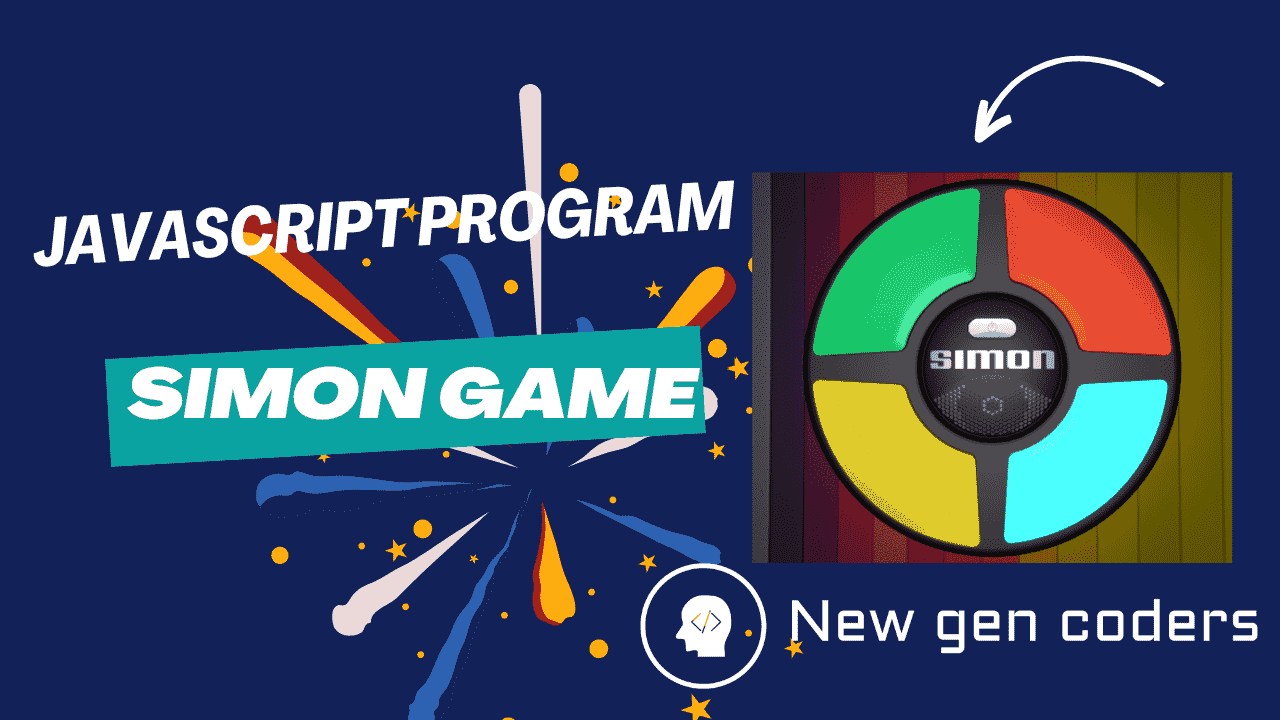
Simon Says Game.
- Jul 21, 2024
- 35
We'll teach you how to use only HTML and CSS to create an interactive 3D image gallery. You can give your website a sleek, dynamic style that wows visitors and improves user experience with just a few lines of code.
How to Design a Tic Tac Toe:
Making a basic HTML framework is the first step towards constructing the gallery. Each image is represented by a div element with a background image.
Let's add CSS styles for the layout, hover interactions, and 3D effect now to spice things up.Using Flexbox to place the images and change their sizes in relation to the viewport dimensions, we will begin with a simple layout.
The magic occurs in the 3D hover effect. An image appears to rotate and zoom in when a user hovers over it, creating the impression of depth.Additionally, we want to confirm that the effect is functional when users click or navigate the gallery with a keyboard.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="wrapper">
<div class="items">
<div class="item" tabindex="0" style="background-image: url(https://picsum.photos/id/1015/400/300)"></div>
<div class="item" tabindex="0" style="background-image: url(https://picsum.photos/id/1021/400/300)"></div>
<div class="item" tabindex="0" style="background-image: url(https://picsum.photos/id/1035/400/300)"></div>
<div class="item" tabindex="0" style="background-image: url(https://picsum.photos/id/1043/400/300)"></div>
<div class="item" tabindex="0" style="background-image: url(https://picsum.photos/id/1050/400/300)"></div>
<div class="item" tabindex="0" style="background-image: url(https://picsum.photos/id/1062/400/300)"></div>
<div class="item" tabindex="0" style="background-image: url(https://picsum.photos/id/1074/400/300)"></div>
<div class="item" tabindex="0" style="background-image: url(https://picsum.photos/id/1080/400/300)"></div>
<div class="item" tabindex="0" style="background-image: url(https://picsum.photos/id/1084/400/300)"></div>
<div class="item" tabindex="0" style="background-image: url(https://picsum.photos/id/237/400/300)"></div>
<div class="item" tabindex="0" style="background-image: url(https://picsum.photos/id/258/400/300)"></div>
<div class="item" tabindex="0" style="background-image: url(https://picsum.photos/id/259/400/300)"></div>
</div>
</div>
</body>
</html>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
:root{
--index: calc(1vw + 1vh);
--transition: cubic-bezier(.1, .7, 0, 1);
}
body{
background-color: #141414;
}
.wrapper{
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
}
.items{
display: flex;
gap: 0.4rem;
perspective: calc(var(--index) * 35);
}
.item{
width: calc(var(--index) * 3);
height: calc(var(--index) * 12);
background-color: #222;
background-size: cover;
background-position: center;
cursor: pointer;
filter: grayscale(1) brightness(.5);
transition: transform 1.25s var(--transition), filter 3s var(--transition), width 1.25s var(--transition);
will-change: transform, filter, rotateY, width;
}
.item::before, .item::after{
content: '';
position: absolute;
height: 100%;
width: 20px;
right: calc(var(--index) * -1);
}
.item::after{
left: calc(var(--index) * -1);
}
.items .item:hover{
filter: inherit;
transform: translateZ(calc(var(--index) * 10));
}
/*Right*/
.items .item:hover + *{
filter: inherit;
transform: translateZ(calc(var(--index) * 8.5)) rotateY(35deg);
z-index: -1;
}
.items .item:hover + * + *{
filter: inherit;
transform: translateZ(calc(var(--index) * 5.6)) rotateY(40deg);
z-index: -2;
}
.items .item:hover + * + * + *{
filter: inherit;
transform: translateZ(calc(var(--index) * 2.5)) rotateY(30deg);
z-index: -3;
}
.items .item:hover + * + * + * + *{
filter: inherit;
transform: translateZ(calc(var(--index) * .6)) rotateY(15deg);
z-index: -4;
}
/*Left*/
.items .item:has( + :hover){
filter: inherit;
transform: translateZ(calc(var(--index) * 8.5)) rotateY(-35deg);
}
.items .item:has( + * + :hover){
filter: inherit;
transform: translateZ(calc(var(--index) * 5.6)) rotateY(-40deg);
}
.items .item:has( + * + * + :hover){
filter: inherit;
transform: translateZ(calc(var(--index) * 2.5)) rotateY(-30deg);
}
.items .item:has( + * + * + * + :hover){
filter: inherit;
transform: translateZ(calc(var(--index) * .6)) rotateY(-15deg);
}
.items .item:active, .items .item:focus {
width: 28vw;
filter: inherit;
z-index: 100;
transform: translateZ(calc(var(--index) * 10));
margin: 0 .45vw;
}
Leave Comments